AngularJS Form Validation
AngularJS Form Validation Tutorial
Form validation is mandatory for any web application. To validate whether the user input is in correct format or not.
RequiredValidator & PatternValidator
1. RequiredValidator
How to use required field validation in angular.
Create a component with the name formval.
c:\>myapp>ng g c formval
2. PatternValidator
PatternValidator is used to validate regex pattern. In the given code pattern validator used to perform email validation.
In the below code, email pattern validator added inside the Validator.
Example:
open up formval.component.ts file and add the code
formval.component.ts
import { Component, OnInit } from '@angular/core'; import { FormGroup, FormControl, Validators, FormBuilder } from '@angular/forms'; import { ReactiveFormsModule } from '@angular/forms'; @Component({ selector: 'app-formval', templateUrl: './formval.component.html', styleUrls: ['./formval.component.css'] }) export class FormvalComponent implements OnInit { requiredForm: any; constructor(private fb: FormBuilder) { this.myForm(); } myForm() { this.requiredForm = this.fb.group({ username: ['', Validators.required ], email: ['', [Validators.required, Validators.pattern("^[a-z0-9._%+-]+@[a-z0-9.-]+\.[a-z]{2,4}$")] ] }); } ngOnInit() { } }
In the above code
We have used form builder to handle all the validation. Constructor is used to create a form with the validation rules.
formval.component.html
<div class="container"> <div class="row"><!-- <p>formval works!</p> --> <h2> Field Required Validation </h2> <form [formGroup] = "requiredForm" novalidate> <div class="form-group"> <label class="center-block">Enter Username: <input class="form-control" formControlName="username"> </label> </div> <div *ngIf="requiredForm.controls['username'].invalid && requiredForm.controls['username'].touched" class="col-sm-6 alert alert-danger"> <div *ngIf="requiredForm.controls['username'].errors.required" class="col-sm-6"> Username is required. </div> </div> <div class="form-group"> <label class="center-block">Email: <input class="form-control" formControlName="email"> </label> </div> <div *ngIf="requiredForm.controls['email'].invalid && requiredForm.controls['email'].touched" class="col-sm-6 alert alert-danger"> <div *ngIf="requiredForm.controls['email'].errors.required" class="col-sm-6"> Email is required. </div> </div> </form> <p>Form value: {{ requiredForm.value | json }}</p> <p>Form status: {{ requiredForm.status | json }}</p> </div> </div>
In the above html code
requiredForm is called global form group object. It is a parent element. Form controls are childrens of requiredForm.
Conditional statement is used to check, if a user has touched the input field but not enter the values then, it displays the error message.
In this form we have created the email control and called email validator.
(You can also try out yourself to perform other types of validators on form controls).
app.component.html
<app-formval> </app-formval> <router-outlet></router-outlet>
Run your application and you could see the below output −
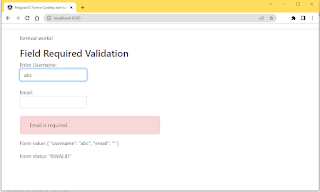
Comments
Post a Comment